Tutorial: Humidity and Temperature Sensor with Arduino
- Posted by Polymath Universata on October 1, 2015 at 12:51pm
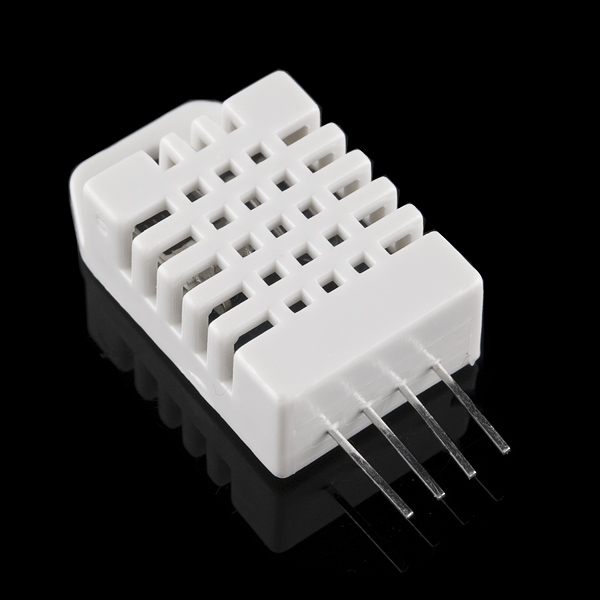
The humidity and temperature sensor RHT03 is an affordable and easy to use sensor. This sensor just need an external resistor to work and you can easily read the data from only one pin. Also, that sensor comes already calibrated.
To use it with your Arduino, you have to download the DHT library here. The files dht.cpp and dht.h are posted in the page, not in a download link. Copy and paste it on a text file in the libraries folder of your IDE.
Reading the RHT03 datasheet, we can check the sensor pinout:

Make the following circuit:

The RHT03 works from 3.3V to 6V, so we will use the 5V provided by the Arduino board. The sensor pin 2 goes to the Arduino pin 5, with a 1Kohm pull-up resistor, like shown in the datasheet:

Open your IDE and paste the code, adapted from here:
#include <dht.h>
dht DHT;
#define DHT22_PIN 5
void setup()
{
Serial.begin(9600);
Serial.println("DHT TEST PROGRAM ");
Serial.print("LIBRARY VERSION: ");
Serial.println(DHT_LIB_VERSION);
Serial.println();
Serial.println("Type,\tstatus,\tHumidity (%),\tTemperature (C)");
}
void loop()
{
// READ DATA
Serial.print("DHT22, \t");
int chk = DHT.read22(DHT22_PIN);
switch (chk)
{
case DHTLIB_OK:
Serial.print("OK,\t");
break;
case DHTLIB_ERROR_CHECKSUM:
Serial.print("Checksum error,\t");
break;
case DHTLIB_ERROR_TIMEOUT:
Serial.print("Time out error,\t");
break;
default:
Serial.print("Unknown error,\t");
break;
}
// DISPLAY DATA
Serial.print(DHT.humidity, 1);
Serial.print(",\t");
Serial.println(DHT.temperature, 1);
delay(1000);
}
If everything went right, you should see something like this on your serial monitor:

References:
http://arduino.cc/playground/Main/DHTLib
http://www.sparkfun.com/products/10167
No comments:
Post a Comment